Introduction
The UNIUYO CSC MATLAB PRACTICAL 1 SOLUTION explain the use of algorithm and flowcharting in details with good clarification to every algorithm problems and the flowchart respectively.
In the vast landscape of computer science and problem-solving, algorithms are the unsung heroes, quietly driving the processes that power our digital world. They are the logical recipes for solving complex problems, serving as the backbone of modern computing. Meanwhile, flowcharts are the visual blueprints that help us understand, plan, and communicate these algorithms.
In this comprehensive content, we will embark on an enlightening journey to explore the twin pillars of algorithm design and flowcharting. Whether you are a budding computer scientist, a seasoned programmer, or someone curious about the inner workings of the digital age, this exploration will equip you with the knowledge and tools to tackle problems methodically and creatively.
An algorithm, in its essence, is a step-by-step set of instructions that, when executed, accomplishes a specific task or solves a particular problem. Think of algorithms as the brilliant minds behind your favorite search engine’s ability to find information in seconds, your GPS’s route-finding prowess, or even the behind-the-scenes magic that powers your social media feed.
But algorithms, by themselves, are not always easy to understand or share. This is where flowcharts come into play. Flowcharts are graphical representations that provide a visual roadmap for understanding and implementing algorithms. These diagrams use symbols and shapes to illustrate the logical sequence of steps, decisions, and processes involved in solving a problem.
Throughout this content, we will unravel the fascinating world of algorithms and flowcharts. We will learn how to design and analyze algorithms, making them efficient and effective. Additionally, we will delve into the art of crafting clear and insightful flowcharts, which are essential for communicating ideas and solutions to others.
UNIUYO CSC MATLAB PRACTICAL 1 SOLUTION 1
Question: Write an Algorithm to convert any number in Gigabyte to Kilobyte
Clarification:
Converting from gigabytes (GB) to kilobytes (KB) involves changing the unit of data storage from one that represents a larger amount to one that represents a smaller amount. Here’s how you can do it:
- Understand the unit conversions:
- 1 gigabyte (GB) is equal to 1,024 megabytes (MB).
- 1 megabyte (MB) is equal to 1,024 kilobytes (KB).
- Calculate the conversion: To convert from gigabytes to kilobytes, you need to multiply by both the number of megabytes in a gigabyte and the number of kilobytes in a megabyte.
- Formula: Kilobytes (KB) = Gigabytes (GB) × 1,024 MB/GB × 1,024 KB/MB
- Plug in the value you want to convert. For example, if you have 2 gigabytes:
- Kilobytes (KB) = 2 GB × 1,024 MB/GB × 1,024 KB/MB
- Calculate the result:
- Kilobytes (KB) = 2,097,152 KB
So, 2 gigabytes is equivalent to 2,097,152 kilobytes. This is because a gigabyte is much larger than a kilobyte, and to get the smaller unit (kilobyte), you need to multiply by the appropriate conversion factors.
1. The Algorithm
Start
Step 1: Enter number in gigabyte
Step 2: Compute number in kilobyte = gigabyte * 1024 * 1024
Step 3: Print Kilobyte
Stop
2. The Flowchart
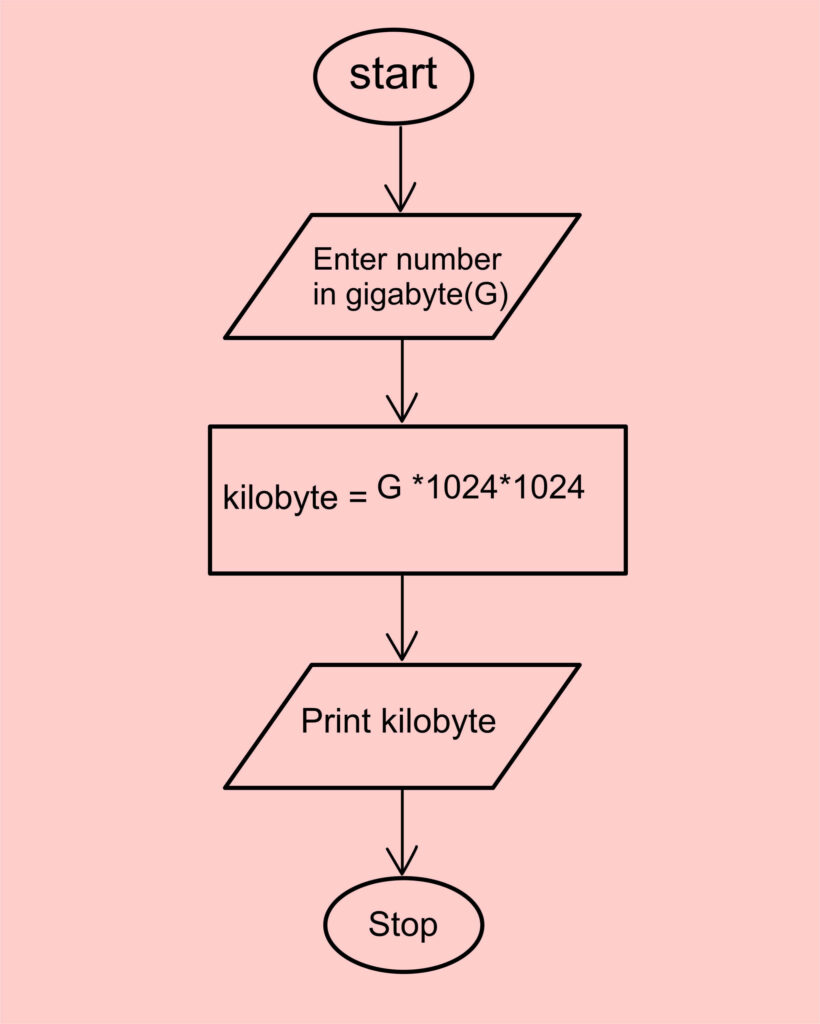
UNIUYO CSC MATLAB PRACTICAL 1 SOLUTION 2
Question: Write an algorithm to find and print the highest number in a list of N numbers
Clarification:
Finding the highest number in a list, often referred to as the maximum or largest element in the list, can be accomplished using various algorithms. One common algorithm for this task is a linear search algorithm, which iterates through the list and keeps track of the current maximum element. Here’s an explanation of the algorithm step by step:
Algorithm to Find the Maximum Number in a List:
a) Initialize Variables:
- Create a variable to store the maximum value found so far. Let’s call it max and initialize it to the first element in
- the array. This initial value ensures that any element in the list will be considered larger than the initial max.
- Start a loop to iterate through the list
b) Iterate Through the List:
- Start a loop that goes through each element in the list one by one.
c) Compare with Current Maximum:
- For each element in the list, compare it with the current max.
- If the current element is greater than the current max, update max with the value of the current element.
d) Continue Iterating:
- Continue this process for all elements in the list, moving through the list one element at a time.
e) Final Result:
- Once the loop finishes, the variable max will contain the maximum value in the list.
f) Return the Maximum Value:
- Return the value of max as the highest number in the list.
3. The Algorithm
Start
Step 1: Input the total number in the List N
Step 2: set an array S[n]
Step 3: initialize max = s[1]
Step 4: Input the first number into s[1]
Step 5: initialize k = 2
Step 6: Is k > N?
Step 7: Yes go to Step 18
Step 8: No
Step 9: Input the next number in s[k]
Step 10: Is Max > s[k]
Step 11: No
Step 12: Max =s[k]
Step 13: k=k+1
Step 14: Go to Step 6
Step 15: Yes:
Step 16: K=K+1
Step 17: Go to Step 6
Step 18: Print Max
Stop
4. The Flowchart
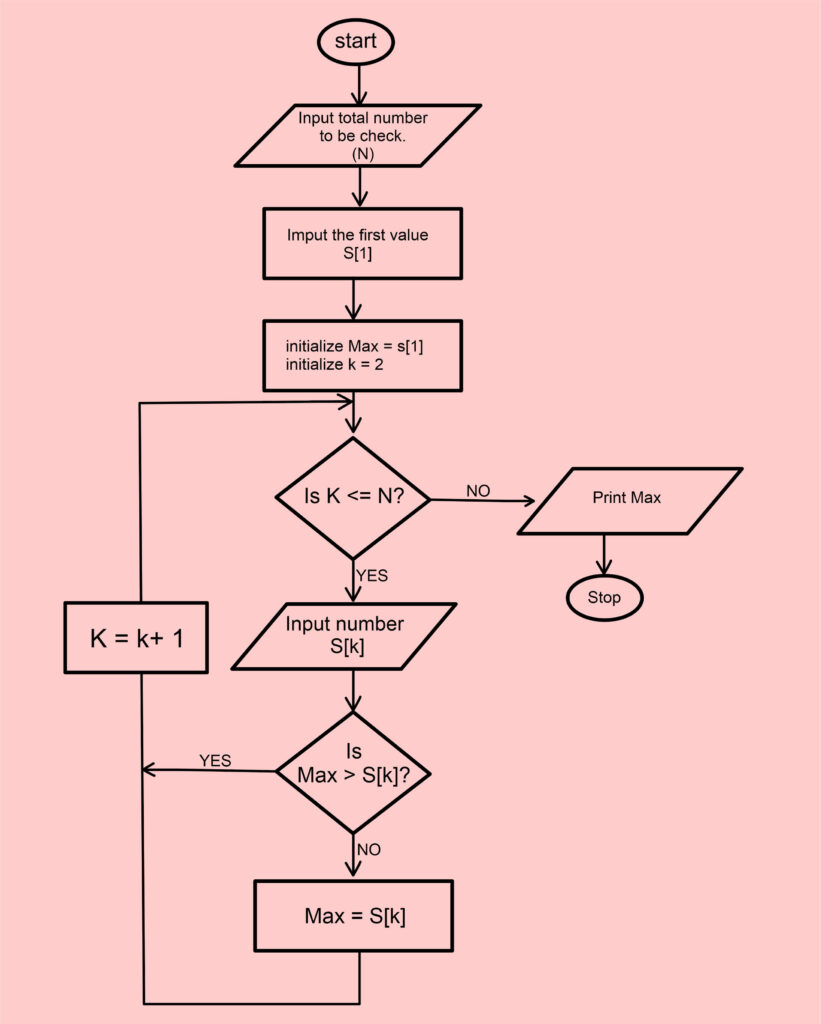
UNIUYO CSC MATLAB PRACTICAL 1 SOLUTION 3
Question: Write an algorithm to compute the product of first 10 odd numbers
Clarification:
To compute the product of the first 10 odd numbers, you can use a simple algorithm that iterates through odd numbers and multiplies them together. Here’s an explanation of the algorithm:
Algorithm to Compute the Product of the First 10 Odd Numbers:
a) Initialize Variables:
- Create a variable to store the product of the odd numbers. Let’s call it product and initialize it to 1.
b) Count and Multiply Odd Numbers:
- Start a loop to iterate through odd numbers. You can do this by initializing a variable number (k) to 1 (the first odd number) and creating a variable count to keep track of how many odd numbers you have multiplied (initialize to 0).
c) Iterate Until You Have the First 10 Odd Numbers:
- In each iteration, multiply the number with the product.
- Increment the count (k) by 1 to keep track of how many odd numbers you have processed.
d) Continue Iterating:
- Continue this process until Count reaches 10, which means you have multiplied the first 10 odd numbers.
e) Final Result:
- The variable product will now contain the product of the first 10 odd numbers.
f) Return the Product:
- Return the value of product as the result.
5. The Algorithm
Start
Step 1: initialize k =
Step 2: initialize Count = 0
Step 3: is count <=10
Step 4: No Go to Step 11
Step 5: Yes
Step 6: Product = Product * k
Step 7: K=k+2
Step 8: Count = count + 1
Step 9: Go to Step 4
Step 10: Print Product
Stop
6. The Flowchart
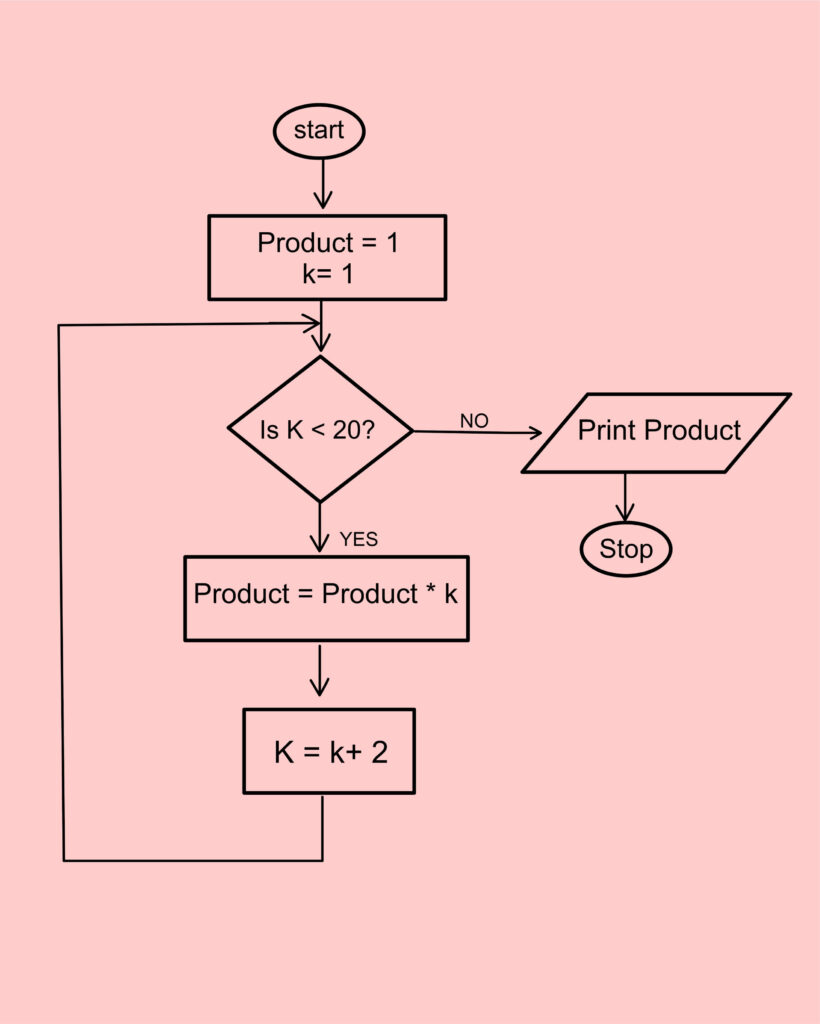
UNIUYO CSC MATLAB PRACTICAL 1 SOLUTION 4
Question: Write an algorithm to get a natural number Q and compute: S = 1+2/4+3/9+…+Q/N
Clarification:
Algorithm to Compute the Sum S:
a) Initialize Variables:
- Create a variable to store the sum and initialize it to 0.
- Create a variable Q to specify the number of terms in the series.
b) Iterate Through Natural Numbers:
- Start a loop to iterate through natural numbers from 1 to Q.
c) Calculate Each Term:
- For each natural number k from 1 to Q, calculate the term k / (k^2).
d) Add the Term to the Sum:
- Add the calculated term to the current value of Sum.
e) Continue Iterating:
- Continue this process for all natural numbers from 1 to Q.
f) Final Result:
- The variable sum will now contain the sum of the series.
g) Return the Sum:
- Return the value of sum as the result.
7. The Algorithm
Start
Step 1: get the value of Q
Step 2: initialize k = 1
Step 3: initialize Sum = 0
Step 4: is k <= Q?
Step 5: No go to Step 11
Step 6: Yes
Step 7: Term = k/(k^2)
Step 8: Sum = Sum + Term
Step 9: K = K+1
Step 10: Go to Step 4
Step 11: Print Sum
Stop
8. The Flowchart
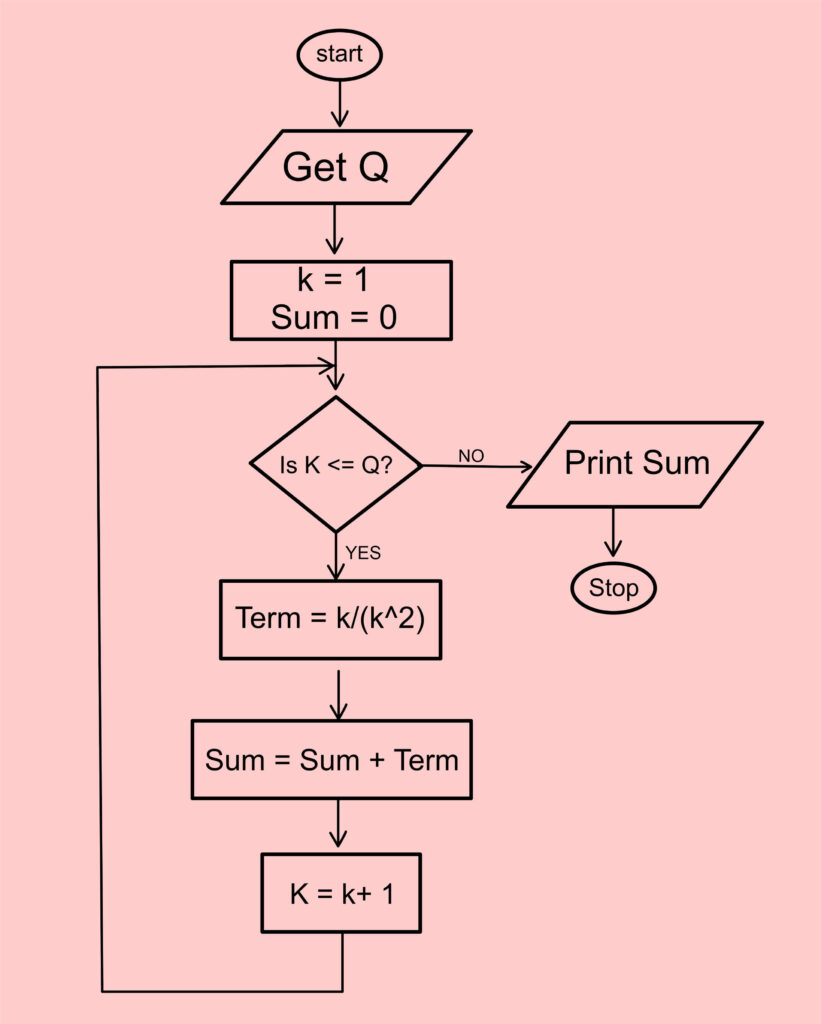
UNIUYO CSC MATLAB PRACTICAL1 SOLUTION 5
Questions: Algorithm to Find the Sum of the Squares of the First 10 Positive Numbers:
Clarification:
a) Initialize Variables:
- Create a variable to store the sum of the squares, which we’ll call sum, and initialize it to 0.
b) Iterate Through Positive Numbers:
- Start a loop to iterate through positive numbers from 1 to 10.
c) Calculate the Square of Each Number:
- For each positive number n from 1 to 10, calculate the square of n, denoted as n^2.
d) Add the Square to the Sum:
- Add the calculated square, n^2, to the current value of sum.
e) Continue Iterating:
- Continue this process for all positive numbers from 1 to 10.
f) Final Result:
- The variable sum will now contain the sum of the squares of the first 10 positive numbers.
g) Return the Sum of Squares:
- Return the value of sum as the result.
9. The Algorithm
Start
Step 1: initialize k=1
Step 2: initialize Sum = 0
Step 3: Sum = Sum + k^2
Step 4: Increment k by 1
Step 5: Is k>10?
Step 6: No Go to Step 3
Step 7: Yes
Step 8: print Sum
Stop
10. The Flowchart
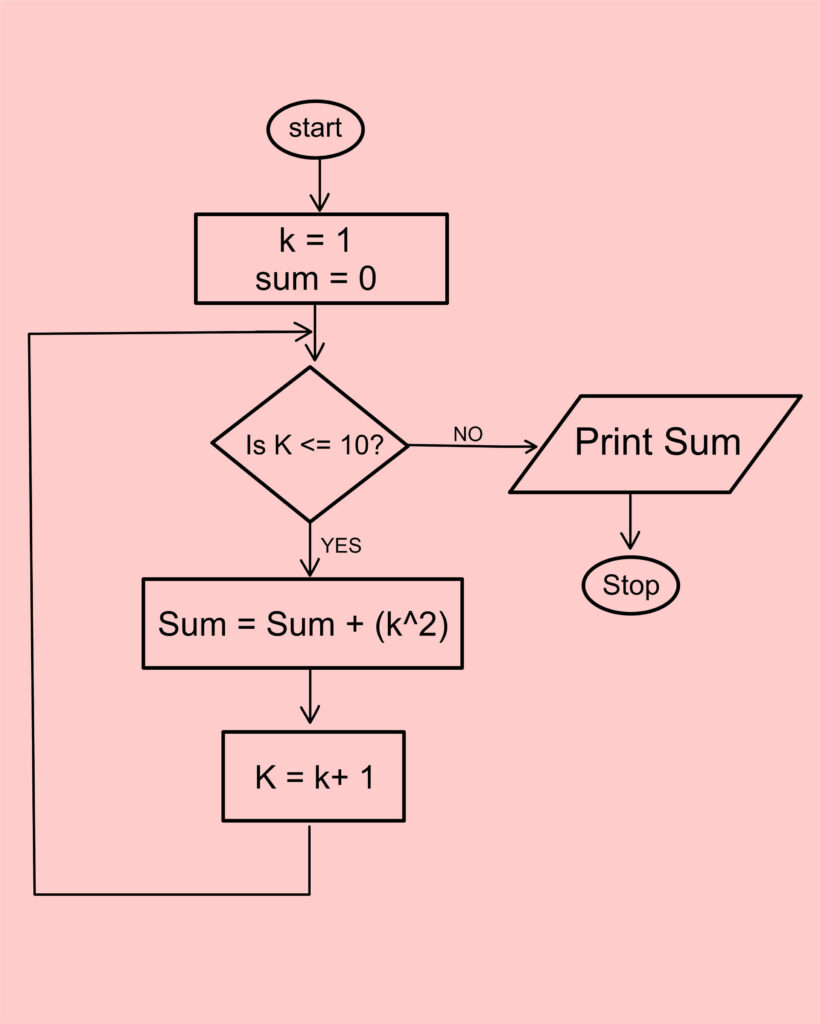
UNIUYO CSC MATLAB PRACTICA 1 SOLUTION 6
Question: Write an algorithm to generate the first 20 elements of the series: 1/3, 2/4, 3/5, 5/7 …
Clarification:
To generate the first 20 elements of the series 1/3, 2/4, 3/5, 4/6, 5/7, you can use an algorithm that iterates through the series, where each element is a fraction of the form “n / (n+2)” where “n” ranges from 1 to 20. Here’s an explanation of the algorithm:
Algorithm to Generate the First 20 Elements of the Series:
a) Initialize Variables:
- Create an empty list to store the elements of the series, which we’ll call series.
b) Iterate Through Numbers from 1 to 20:
- Start a loop to iterate through numbers from 1 to 20.
c) Calculate Each Element:
- For each number n in the range from 1 to 20, calculate the element of the series as n / (n+2).
d) Add the Element to the Series:
- Add the calculated element to the series list.
e) Continue iterating:
- Continue this process for all numbers from 1 to 20.
f) Final Result:
- The series list will now contain the first 20 elements of the series.
g) Return the Series:
- Return the series as the result.
11. The algorithm
Start
Step 1: Set an array Series
Step 2: initialize n = 1
Step 3: Compute the Series[n] = n/(n+2)
Step 4: n = n+1
Step 5: Is n > 20?
Step 6: No Go to Step 3
Step 7: Yes
Step8: Print the series[n]
Stop
12. The flowchart
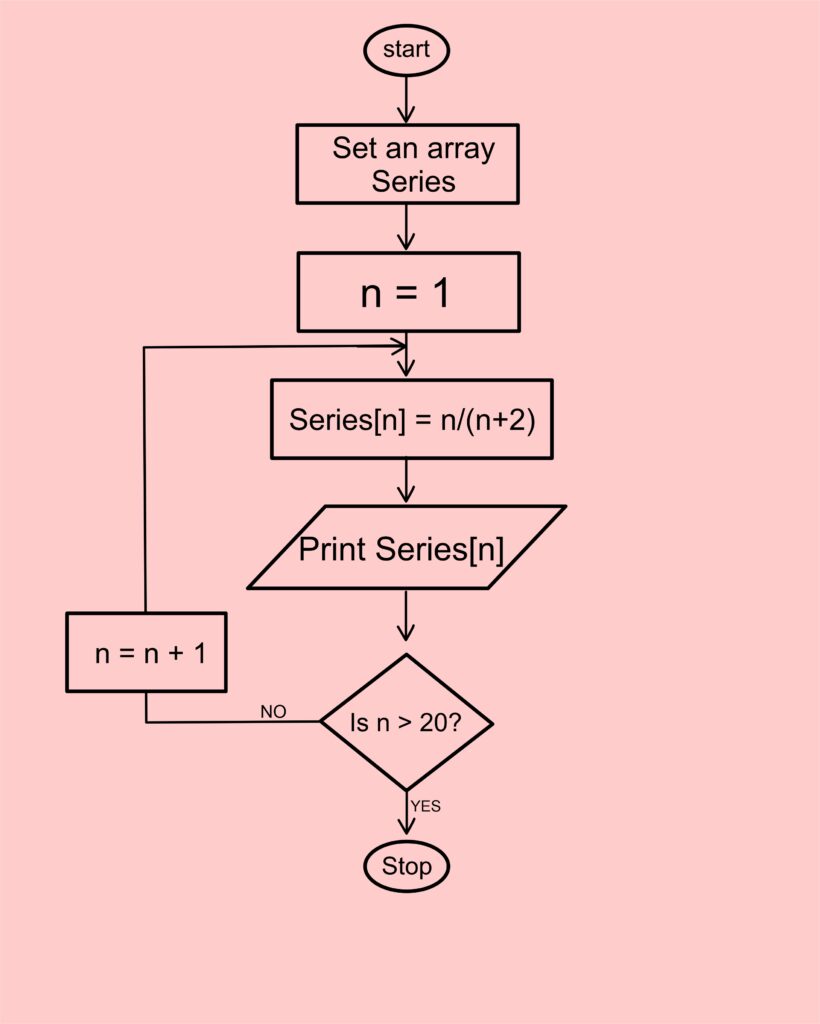
Feedback
Your feedback is incredibly valuable to us! We’re delighted that you found the content on our platform helpful. If you’ve been blessed by the materials and information provided, we would greatly appreciate your comments in the section below.
- Share your thoughts about how the content has benefited you. Your testimonials can motivate fellow students.
- If you have any questions or need further clarification on a topic, don’t hesitate to ask. We’re here to help!
- Your suggestions for improvement or topics you’d like to see covered in the future are always welcome. We strive to make our content as relevant as possible.
Academic Integrity Disclaimer (Please Read cautiously)
Dear Students,
We would like to remind you of the importance of academic integrity and the rules governing examinations and practical assessments. It is against the principles of fair and honest evaluation to copy solutions from this site and use them in the practical or examination halls.
Copying solutions from this site and submitting them as your own work violates the trust we place in you to demonstrate your understanding and skills during assessments. It also contradicts the principles of academic honesty and can result in serious consequences, including academic penalties.
We encourage you to use the resources provided here as a learning aid and reference to enhance your understanding of the subject matter. However, it is essential that you independently work on assignments, practicals, and examinations to showcase your individual knowledge and capabilities.
Please respect the rules and guidelines set forth by your educational institution regarding academic honesty and integrity. Failure to do so may not only harm your academic progress but also tarnish your reputation.
We are here to support your learning journey and provide guidance when needed. If you have any questions or require assistance with understanding the material, please do not hesitate to reach out to us.
Let’s work together to create an environment of trust, respect, and academic excellence.
Sincerely,
Sunday Fola
Find more solutions at webzalo school portal, subscribe to this site to get the latest scholarship update and you can be updated with our top info on the current trends in the world.