UNIUYO JAVA LABWORK 2 (PROJECT 1)
Write a Java program to implement the current students’ grading system. Your program should accept the test and examination score, calculate the total and determine the grade for n number of students. The output on the screen should be in a tabular form; Reg_Num, Test, Exam, Total and grade.
Solution

Explanation
This Java program allows users to input test and exam scores for a given number of students and calculates their total scores and grades based on predefined grade boundaries. Let’s break down the code:
- import java.util.Scanner;: Imports the Scanner class from the java.util package to allow user input.
- public class Project11 {: Defines a class named Project11.
- public static void main(String[] args) {: Defines the main method, which is the entry point of the program.
- Scanner sc=new Scanner(System.in);: Creates a new Scanner object named sc to read input from the console.
- System.out.print(“Pls enter the total number of students: “);: Prompts the user to enter the total number of students.
- int stud=sc.nextInt();: Reads the total number of students entered by the user and stores it in the variable stud.
- String[] RegNo=new String[stud];: Declares an array named RegNo to store the registration numbers of the students.
- int [] Test =new int[stud];: Declares an array named Test to store the test scores of the students.
- int[]Exam = new int[stud];: Declares an array named Exam to store the exam scores of the students.
- int [] Total = new int[stud];: Declares an array named Total to store the total scores of the students.
- String[] Grade= new String[stud];: Declares an array named Grade to store the grades of the students.
- for (int i=0; i<stud; i++) { … }: Iterates over each student to input their registration number, test score, and exam score.
- System.out.println();: Prints a newline for formatting purposes.
- System.out.print(“Enter Student “+(i+1)+ ” Reg number: “);: Prompts the user to enter the registration number of the current student.
- RegNo[i]=sc.next();: Reads the registration number entered by the user and stores it in the RegNo array.
- System.out.print(“Enter the test score for “+RegNo[i]+ “: “);: Prompts the user to enter the test score for the current student.
- Test[i]=sc.nextInt();: Reads the test score entered by the user and stores it in the Test array.
- System.out.print(“Enter the exam score for “+RegNo[i]+ “: “);: Prompts the user to enter the exam score for the current student.
- Exam[i]=sc.nextInt();: Reads the exam score entered by the user and stores it in the Exam array.
- Total[i]=Test[i]+Exam[i];: Calculates the total score for the current student by adding their test and exam scores.
- System.out.println(“\n\nSN\tREG NO\t\tTEST\tEXAM\tTOTAL\tGRADE”);: Prints a header for the table displaying student information.
- for (int i=0; i<stud; i++) { … }: Iterates over each student to calculate their grade based on their total score and prints their information.
- if(Total[i]>=70) { … } else if …: Determines the grade for each student based on their total score using predefined grade boundaries.
- System.out.println((i+1)+”\t”+RegNo[i]+”\t”+Test[i]+”\t”+Exam[i]+”\t”+Total[i]+”\t”+Grade[i]) ;: Prints the student’s information, including their serial number, registration number, test score, exam score, total score, and grade.
Output

UNIUYO JAVA LABWORK 2 (PROJECT 2)
The management board of De-Gibson University decided to automate the implementation of the board’s decision on increasing the school fees for next academic session, which depends on the number of students admitted given a cut off mark of 275; that is, those admitted is above 60% of total applicants. write a Java program that will determine the number of candidates admitted or not admitted, and advise the management whether to increase the school fees or not.
Solution

Explanation
This Java program simulates a scenario where applicants are considered for admission based on their scores. Let’s go through the code line by line:
- import java.util.Scanner;: Imports the Scanner class from the java.util package to allow user input.
- public class PROJECT12 {: Defines a class named PROJECT12.
- public static void main(String[] args) {: Defines the main method, which is the entry point of the program.
- Scanner sc = new Scanner(System.in);: Creates a new Scanner object named sc to read input from the console.
- System.out.print(“Pls enter the total number of applicants: “);: Prompts the user to enter the total number of applicants.
- int app = sc.nextInt();: Reads the total number of applicants entered by the user and stores it in the variable app.
- System.out.print(“Pls enter the total number of students that scored above 275: “);: Prompts the user to enter the total number of students who scored above 275.
- int add = sc.nextInt();: Reads the total number of students who scored above 275 entered by the user and stores it in the variable add.
- int admitted = add;: Initializes the variable admitted with the value of add, representing the number of students admitted.
- int NotAdmitted = app – add;: Calculates the number of students not admitted by subtracting the number admitted (add) from the total number of applicants (app).
- double Decision = (add * 100) / app;: Calculates the percentage of students admitted by dividing the number admitted (add) by the total number of applicants (app) and multiplying by 100.
- if (Decision > 60) { … } else { … }: Checks if the percentage of students admitted is greater than 60%. If it is, the program prints a message indicating that school fees should be increased. Otherwise, it prints a message indicating that school fees should not be increased.
- System.out.println(“\nTotal Number Admitted: ” + admitted … );: Prints the total number of students admitted, the total number not admitted, the percentage of students admitted, and the decision based on the admission percentage.
Output


UNIUYO JAVA LABWORK 2 (PROJECT 3)
Write a Java program that requires the user to enter a single character from the alphabet, print Vowel or consonant, depending on the user input. If the user input is not a letter between Aa – Zz, print an error message.
Solution
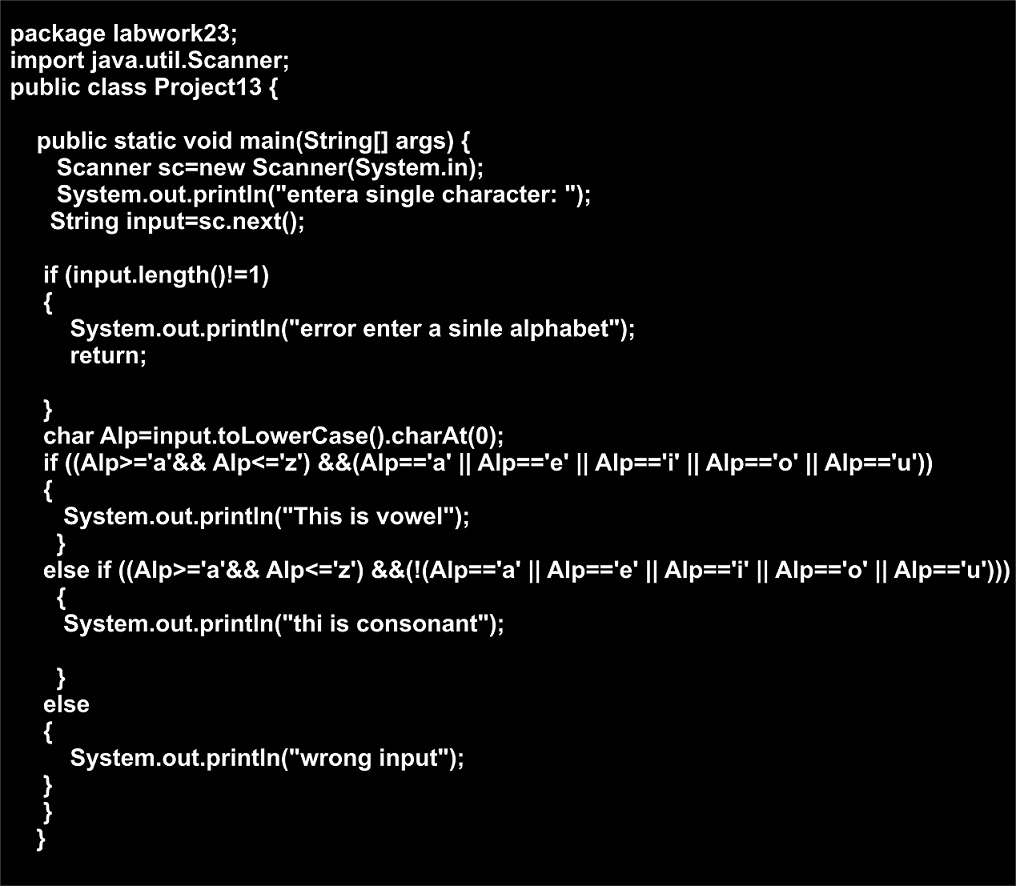
Explanation
- package labwork23;: Specifies the package name where the class Project13 is located.
- import java.util.Scanner;: Imports the Scanner class from the java.util package to allow user input.
- public class Project13 {: Defines a class named Project13.
- public static void main(String[] args) {: Defines the main method, which is the entry point of the program.
- Scanner sc = new Scanner(System.in);: Creates a new Scanner object named sc to read input from the console.
- System.out.println(“Enter a single character: “);: Prompts the user to enter a single character.
- String input = sc.next();: Reads the user input and stores it in the input variable.
- if (input.length() != 1) { … }: Checks if the length of the input string is not equal to 1, indicating that the user did not enter a single character.
- char Alp = input.toLowerCase().charAt(0);: Converts the input string to lowercase and extracts the first character, storing it in the variable Alp.
- if ((Alp >= ‘a’ && Alp <= ‘z’) && (Alp == ‘a’ || Alp == ‘e’ || Alp == ‘i’ || Alp == ‘o’ || Alp == ‘u’)) { … }: Checks if Alp is a lowercase letter and if it is one of the vowels (‘a’, ‘e’, ‘i’, ‘o’, ‘u’).
- else if ((Alp >= ‘a’ && Alp <= ‘z’) && (!(Alp == ‘a’ || Alp == ‘e’ || Alp == ‘i’ || Alp == ‘o’ || Alp == ‘u’))) { … }: Checks if Alp is a lowercase letter and not one of the vowels, indicating that it is a consonant.
- else { … }: Handles the case where the input is not a letter.
Output

UNIUYO JAVA LABWORK 2 (PROJECT 4)
Write a Java to generate 10 terms of the sequence 1/2, 1/4, 1/6, 1/8, 1/10, …, 1/M. Your outputs must be in fraction (e.g. 1/2).
Solution
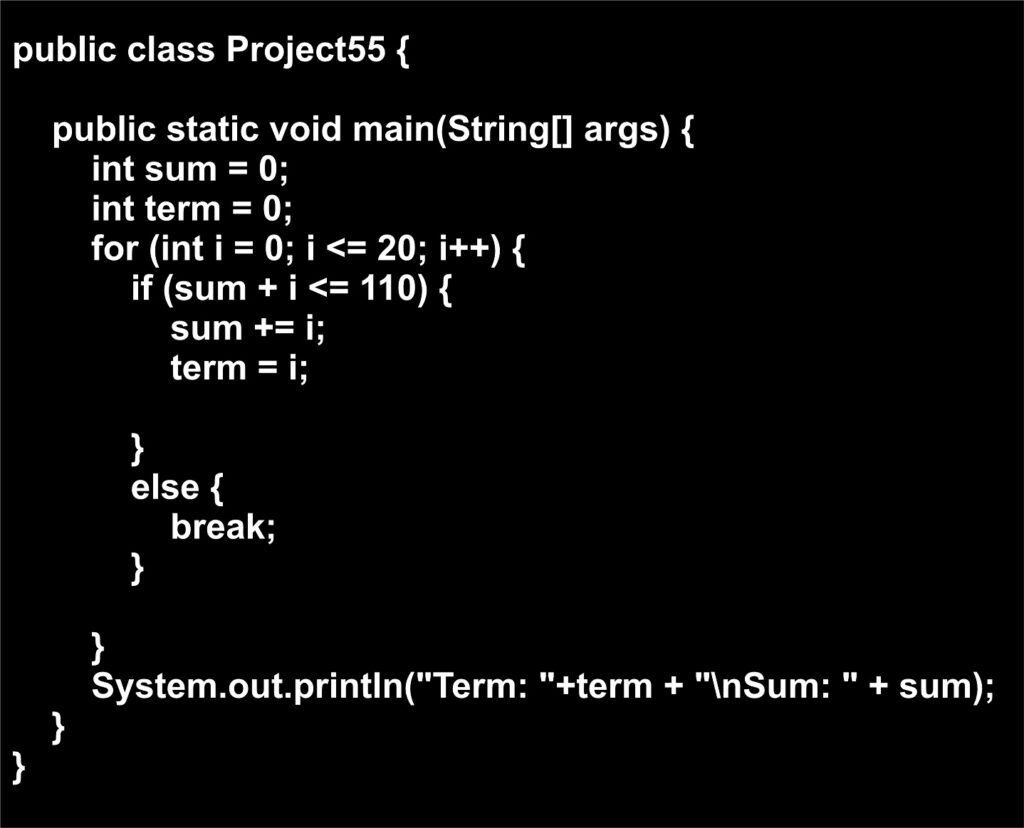
Explanation
- public class Project44 {: Defines a class named Project44.
- public static void main(String[] args) {: Defines the main method, which is the entry point of the program.
- System.out.println(“enter the last number in the series: “);: Prints a message asking the user to enter the last number in the series.
- Scanner sc = new Scanner(System.in);: Creates a new Scanner object named sc to read input from the console.
- int lastNumber = sc.nextInt();: Reads the last number in the series entered by the user and stores it in the variable lastNumber.
- System.out.print(“The series are: “);: Prints a message indicating that the series will be displayed.
- for (int i = 2; i <= lastNumber; i += 2) { … }: Starts a loop that iterates over even numbers from 2 up to the last number in the series entered by the user.
- System.out.print(“1/” + i + “, “);: Prints each term of the series in the format “1/x,” where “x” represents the current even number in the series.
Output
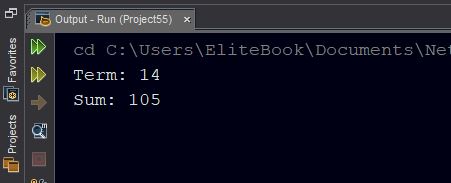
UNIUYO JAVA LABWORK 2 (PROJECT 5)
Write a Java program to add natural numbers between 1 to 20 but ensuring that the final sum of these numbers does not exceed 110. Display the final sum and the nth term of the natural number.
Solution
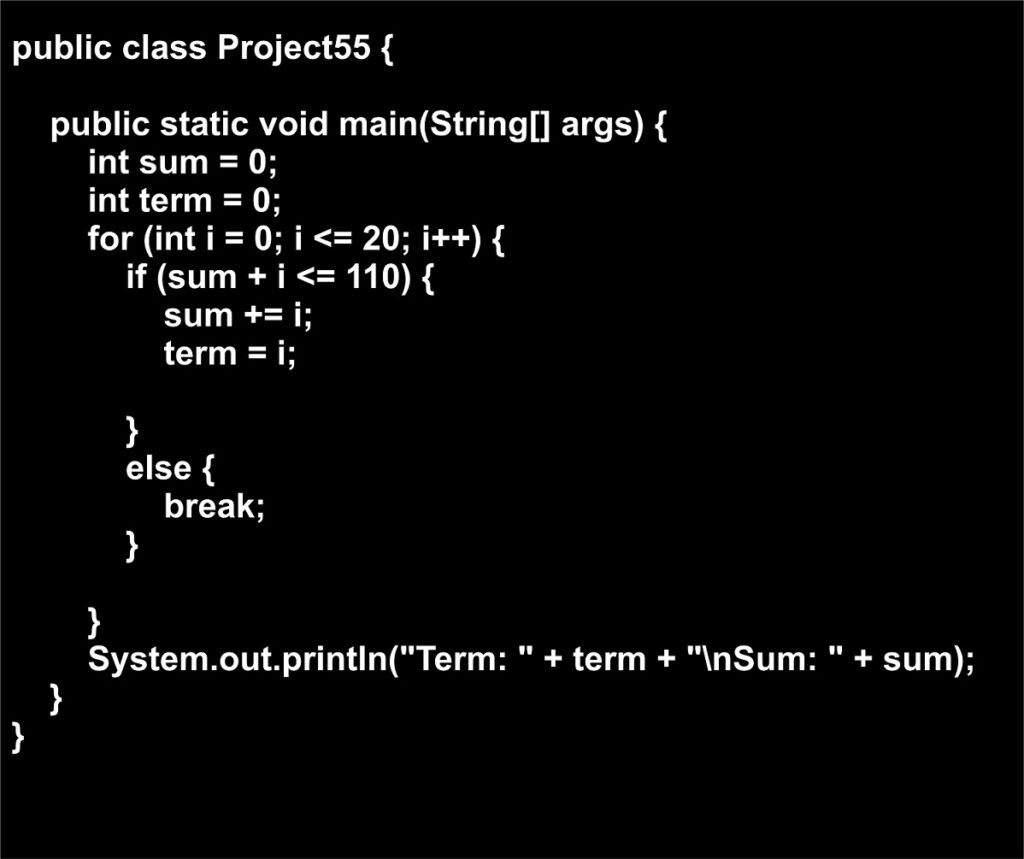
Explanation
- public class Project55 {: Defines a class named Project55.
- public static void main(String[] args) {: Defines the main method, which is the entry point of the program.
- int sum = 0;: Initializes a variable sum to store the sum of the terms.
- int term = 0;: Initializes a variable term to store the value of the last term added to the sum.
- for (int i = 0; i <= 20; i++) { … }: Starts a loop that iterates from 0 up to 20.
- if (sum + i <= 110) { … }: Checks if adding the current value of i to the sum (sum + i) will not exceed 110.
- sum += i;: If the condition is met, adds the current value of i to the sum.
- term = i;: Updates the term variable with the current value of i.
- else { break; }: If adding the current value of i would exceed 110, breaks out of the loop.
- System.out.println(“Term: ” + term + “\nSum: ” + sum);: Prints the value of the last term added (term) and the total sum (sum).
Output
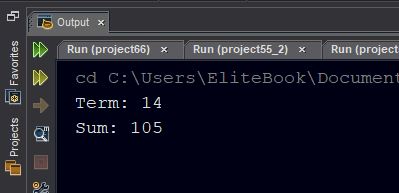
UNIUYO JAVA LABWORK 2 (PROJECT 6)
A farming industry is in need of a program to select Irish apples after harvesting as green-apple, and red-apples. The green-apples are identified as even numbers (2,4,6,…), And the red-apples as odd number (1,3,5,…). However, any apple identified as any number divisible by five is defective and hence should not be selected. Write a Java program as a prototype to solve this problem assuming that n number of Irish apples were harvested. Hint: your program should output a set of even and odd numbers.
Solution 1
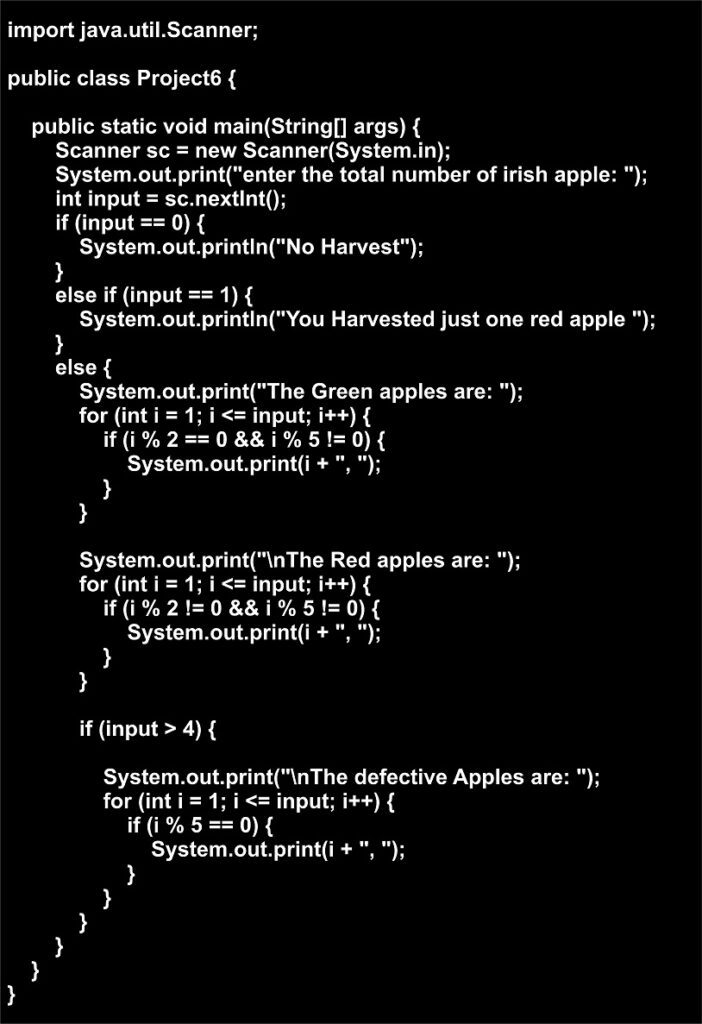
Explanation
- import java.util.Scanner;: Imports the Scanner class from the java.util package to allow user input.
- public class Project66 {: Defines a class named Project66.
- public static void main(String[] args) {: Defines the main method, the entry point of the program.
- Scanner sc = new Scanner(System.in);: Creates a Scanner object named sc to read input from the console.
- System.out.print(“enter the total number of irish apple: “);: Prompts the user to enter the total number of Irish apples.
- int input = sc.nextInt();: Reads the integer input entered by the user and stores it in the variable input.
- if (input == 0) { … }: Checks if the input is zero. If true, prints “No Harvest”.
- else if (input == 1) { … }: Checks if the input is one. If true, prints “You Harvested just one red apple”.
- else { … }: If the input is neither zero nor one, proceeds with the following logic.
- for (int i = 1; i <= input; i++) { … }: Starts a loop to iterate from 1 up to the value of input.
- if (i % 2 == 0 && i % 5 != 0) { … }: Checks if the current number (i) is even and not divisible by 5. If true, prints it as a green apple.
- System.out.print(“\nThe Red apples are: “);: Prints a label for the red apples.
- if (input > 4) { … }: Checks if the input is greater than 4. If true, proceeds with the following logic.
- System.out.print(“\nThe defective Apples are: “);: Prints a label for the defective apples.
Output
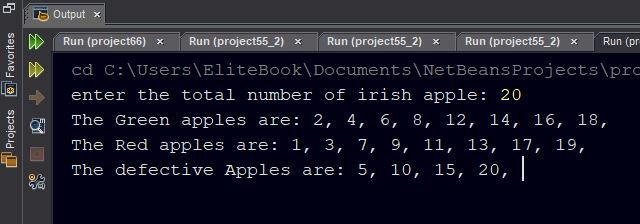
Solution 2 (Accepting the apple number from user)

Explanation
- import java.util.Scanner;: Imports the Scanner class from the java.util package to allow user input.
- public class Project55_2 {: Defines a class named Project55_2.
- public static void main(String[] args) {: Defines the main method, the entry point of the program.
- Scanner sc = new Scanner(System.in);: Creates a Scanner object named sc to read input from the console.
- System.out.println(“Enter the total number of Irish apple harvested”);: Prompts the user to enter the total number of Irish apples harvested.
- int input = sc.nextInt();: Reads the integer input entered by the user and stores it in the variable input.
- int Harvest[] = new int[input];: Creates an integer array named Harvest to store the details of each apple, with the size determined by the user input.
- int green = 0; int red = 0; int defect = 0;: Initializes variables to count the number of green, red, and defective apples.
- for (int i = 0; i < input; i++) { … }: Starts a loop to iterate from 0 up to the value of input.
- System.out.print(“enter the irish apple ” + (i + 1) + ” number: “);: Prompts the user to enter the details of each apple.
- Harvest[i] = sc.nextInt();: Reads the integer input entered by the user and stores it in the Harvest array at index i.
- if (Harvest[i] % 2 == 0 && Harvest[i] % 5 != 0) { … }: Checks if the current apple is green based on its properties and increments the green counter if true.
- else if (Harvest[i] % 2 != 0 && Harvest[i] % 5 != 0) { … }: Checks if the current apple is red based on its properties and increments the red counter if true.
- else if (Harvest[i] % 5 == 0) { … }: Checks if the current apple is defective based on its properties and increments the defect counter if true.
- if (green != 0) { … }: Checks if there are any green apples and prints them if true.
- if (red != 0) { … }: Checks if there are any red apples and prints them if true.
- if (defect != 0) { … }: Checks if there are any defective apples and prints them if true.
Output
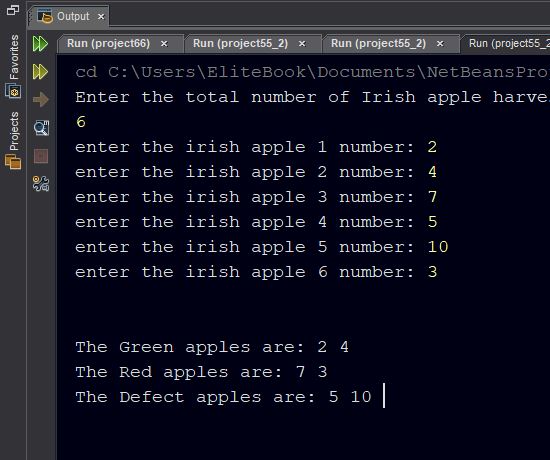
UNIUYO JAVA LABWORK 2 (PROJECT 7a)

Solution

Output

UNIUYO JAVA LABWORK 2 (PROJECT 7b)

Solution

Output

UNIUYO JAVA LABWORK 2 (PROJECT 8)
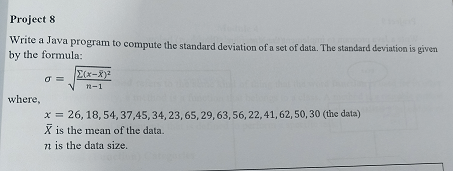
Solution

Output
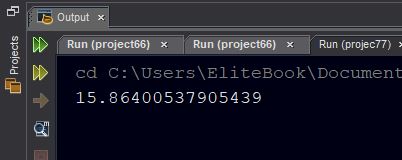
UNIUYO JAVA LABWORK 2 (PROJECT 9)

Solution
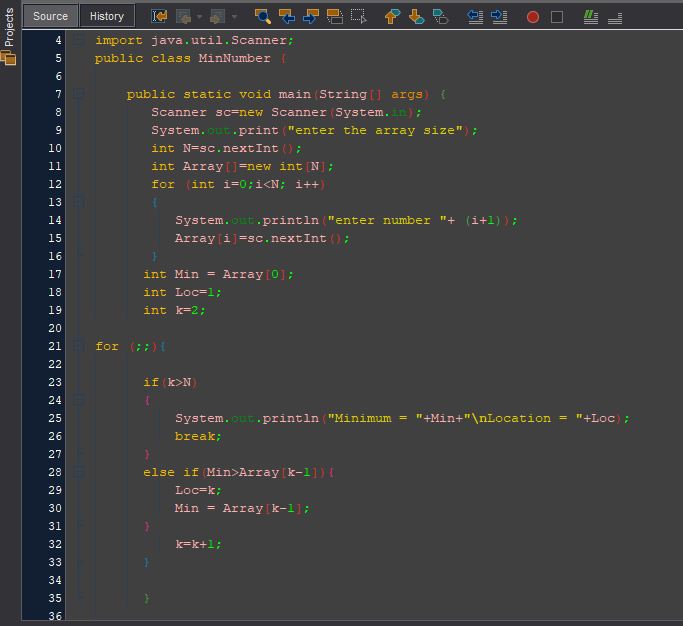
Output

Disclaimer
The solutions provided for the Java lab work are intended solely for study purposes. Under no circumstances should any student directly copy these solutions into the exam or test hall. These solutions are meant to enhance understanding and facilitate learning, and students are encouraged to use them responsibly as study guides. Plagiarism or academic dishonesty is strictly prohibited and can result in severe consequences, including disciplinary action. It is essential for students to demonstrate their understanding of the concepts independently during assessments.
Study more on java projects here